Understanding Asynchronous Programming in JavaScript
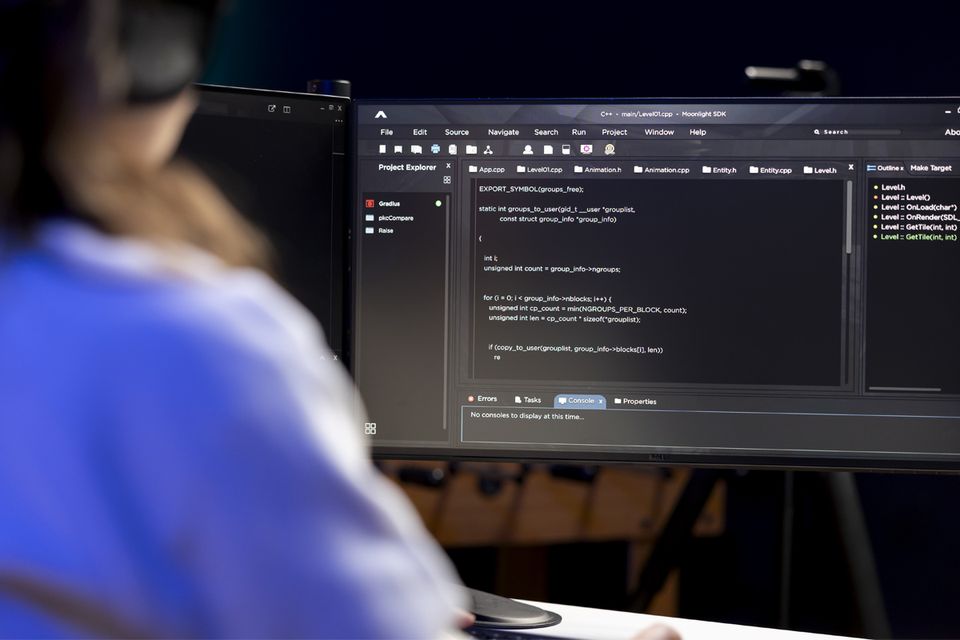
JavaScript is one of the most widely used languages for building interactive and responsive web applications. One of the most powerful features of JavaScript is its ability to handle asynchronous operations—allowing programs to remain responsive, efficient, and fast, even while performing time-consuming tasks in the background. In this article, we’ll explore the concept of asynchronous programming in JavaScript, its importance, and the modern approaches used to manage it.
What is Asynchronous Programming?
Asynchronous programming refers to a method of writing code that allows tasks to run in the background without blocking the main thread. In simpler terms, it lets your application continue running while it waits for a certain operation—like fetching data from a server or reading a file—to complete.
JavaScript runs in a single-threaded environment, which means it can only do one thing at a time. Without asynchronous programming, every task would have to be completed before moving on to the next. This would create major delays, especially when dealing with tasks that take time to finish. Asynchronous programming solves this problem by allowing these tasks to run independently of the rest of the program.
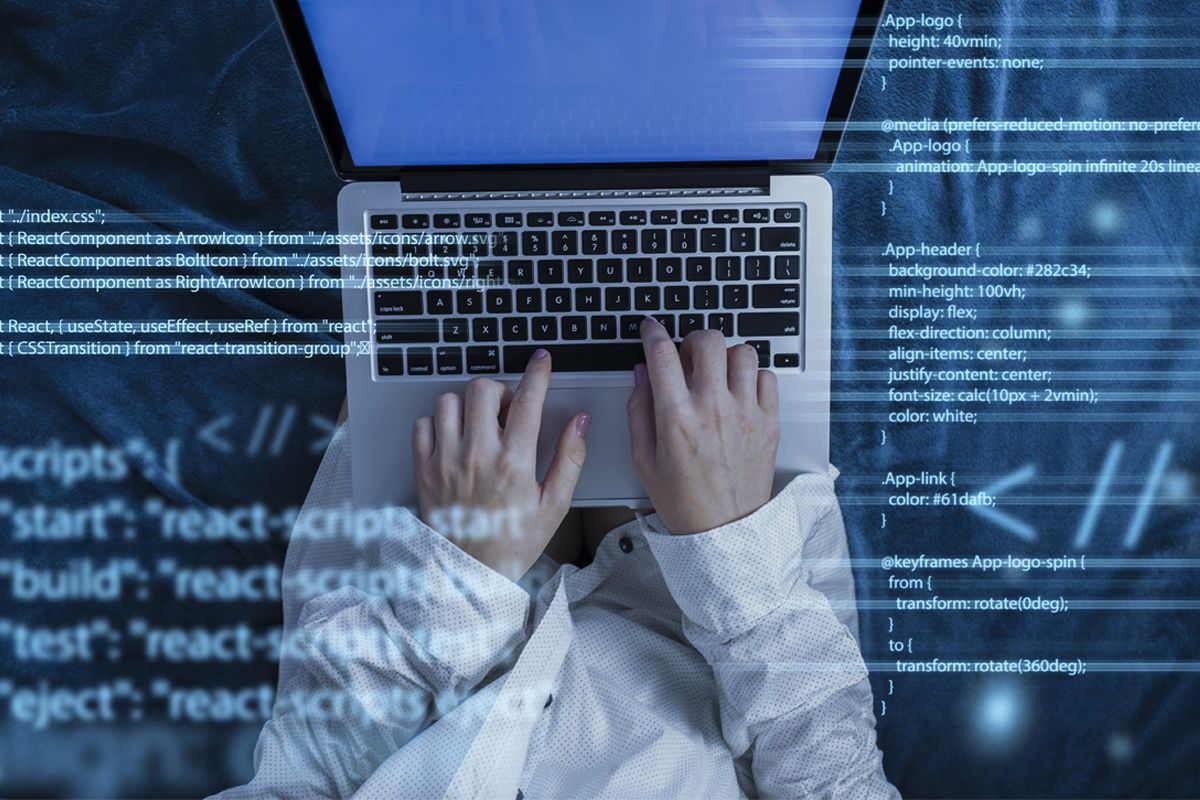
Why Asynchronous Programming Matters
Modern web applications require smooth and dynamic user experiences. Imagine filling out a form or scrolling a page while a background process loads data. Without asynchronous capabilities, users would experience lag or frozen interfaces. Asynchronous programming allows developers to create non-blocking experiences where the interface stays responsive, even when the app is waiting for data or performing complex tasks.
Some of the key benefits include:
- Improved application performance
- Better user experience
- Efficient use of system resources
- Support for real-time interactions
The Evolution of Asynchronous JavaScript
As JavaScript has matured, so have the ways developers handle asynchronous behavior. Initially, developers used callbacks, which involved passing a function to be executed after a task completes. While effective, callbacks often resulted in deeply nested and difficult-to-read code, sometimes referred to as "callback hell."
To address this challenge, JavaScript introduced Promises—a cleaner and more structured way to handle asynchronous events. Promises allow developers to write more linear and readable code, with built-in support for error handling.
The latest and most developer-friendly evolution is the use of async/await, introduced in ECMAScript 2017. This approach allows developers to write asynchronous code that looks and behaves like synchronous code, significantly improving readability and reducing complexity.
Where Asynchronous Programming Shines
Asynchronous programming is essential in many real-world scenarios:
- Web APIs: Applications often need to fetch data from a server. Async code allows the app to load this data without freezing the interface.
- User authentication: Login processes involve checking user credentials against a server. Asynchronous code handles this without delay.
- Real-time features: Applications like chat apps, notifications, or live dashboards rely on background updates.
- File handling: Uploading or downloading files is often time-consuming, and async logic ensures the UI remains responsive during these operations.
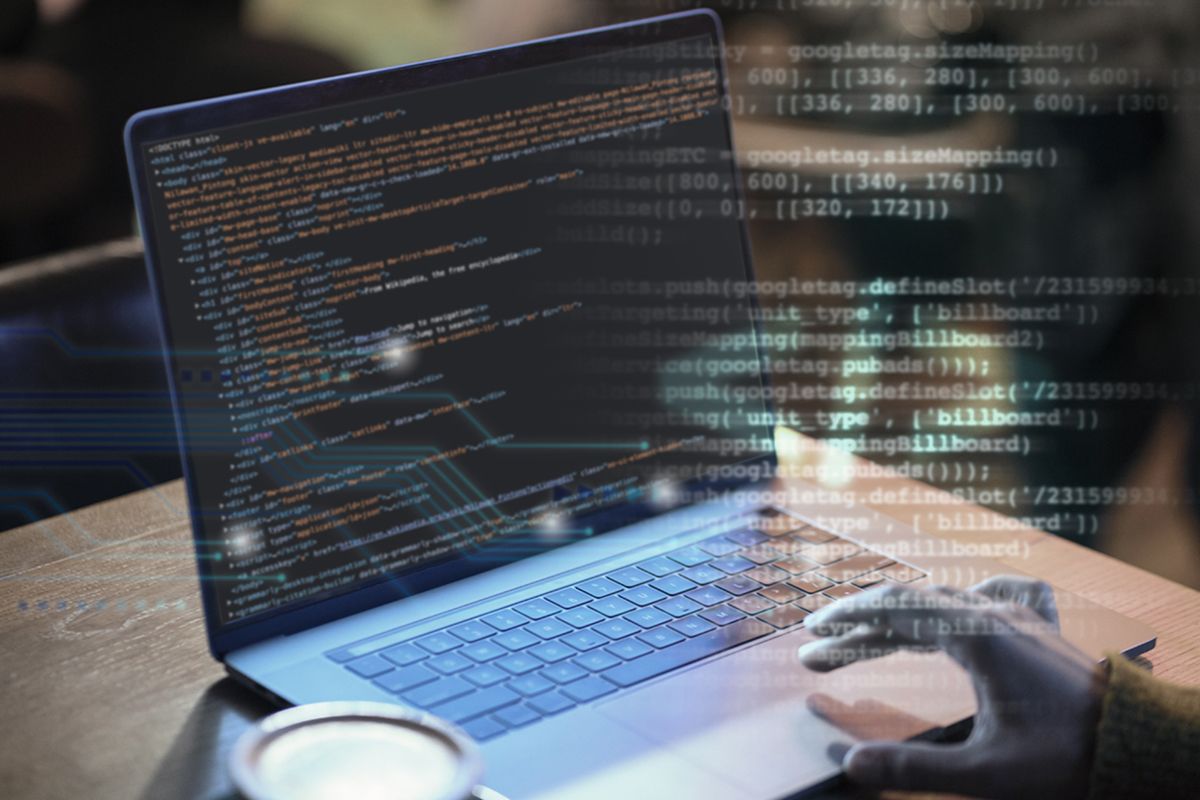
Challenges to Consider
While asynchronous programming brings many benefits, it also comes with its own set of challenges:
- Debugging: Errors in async code can be harder to trace than in synchronous logic.
- State management: When multiple tasks run simultaneously, keeping track of application state becomes more complex.
- Error handling: Asynchronous operations require a careful approach to catch and manage potential failures properly.
Despite these challenges, the advantages of asynchronous programming far outweigh the downsides when implemented thoughtfully.
Best Practices
To make the most of asynchronous programming in JavaScript, developers should follow a few best practices:
1. Use async/await where possible – It improves code readability and maintains flow similar to synchronous operations.
2. Handle errors gracefully – Implement proper error handling to catch and resolve issues without affecting user experience.
3. Avoid nested callbacks – Stick to structured patterns like promises and async functions to avoid complexity.
4. Think in terms of workflows – Break tasks into logical steps and handle each step asynchronously to maintain clean and modular code.
5. Test and debug – Use appropriate tools to monitor asynchronous behavior and ensure everything runs as expected.
Final Thoughts
Asynchronous programming is no longer an advanced concept—it’s a core skill for any JavaScript developer. From user interactions to background data fetching, it powers the interactivity and responsiveness of modern applications. Understanding how to implement async logic effectively will not only improve your applications but also help you write more maintainable, high-performance code.
Whether you're building a single-page application or a full-stack platform, mastering asynchronous programming in JavaScript will elevate your development skills and prepare you to meet the demands of today’s fast-paced digital world.